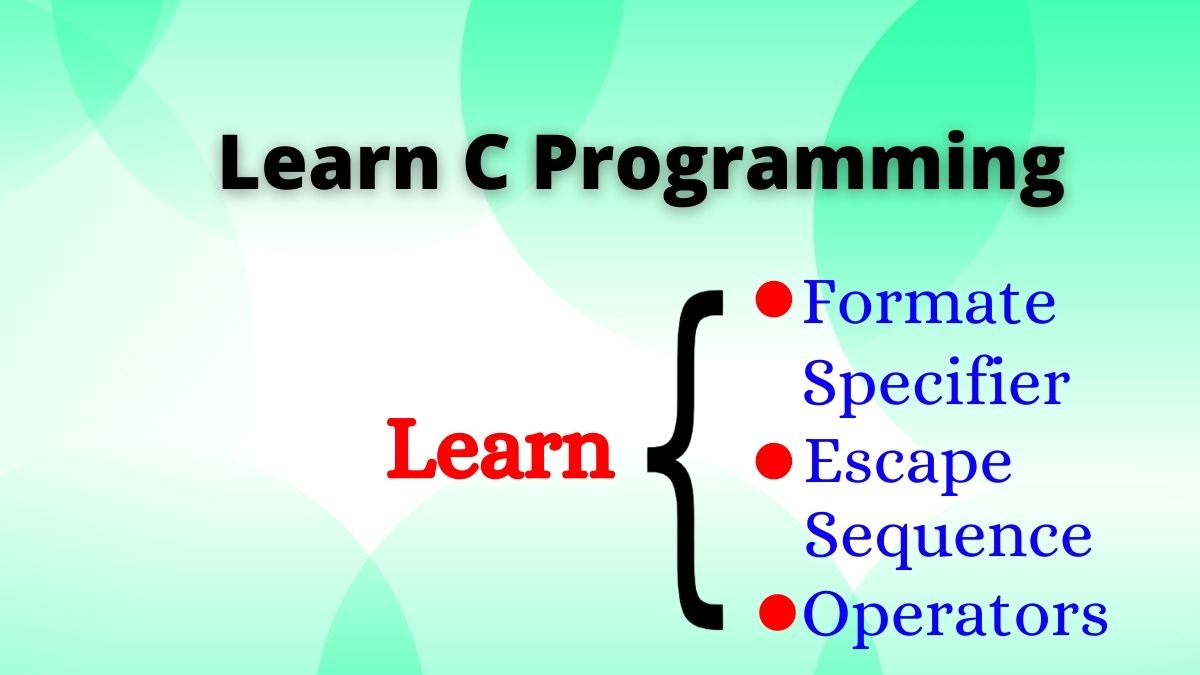
Operators
An operators is a symbol used to perform oprations in given programming language.
Types of Operators:
01)Arithmetic operators
02)Relational operators
03)Logical operators
04)Bitwise operators
05)Assignment operators
`Rightarrow` Explains All Operators
01)Arithmetic operators
Operators | Description | Examples |
---|---|---|
+ | Addition | 3 + 4 = 7 |
- | Substration | 3 - 3 = 0 |
* | Multiplication | 3 * 5 = 15 |
/ | Division | 15 / 3 = 3 |
% | Modulus | 8 % 2 (Reminder) = 2 |
If value float then answer is float then use %f
Here the output is:
a + b = 40
a - b = 28
a * b = 204
a / b = 5
a b = 4
02)Relational operators
Operators | Description | Examples | Results |
---|---|---|---|
= = | Is equal to | 5 == 2 | False |
!= | Is not equal to | 5 != 2 | True |
> | Greater than | 5 > 2 | True |
< | Less than | 5 < 2 | False |
>= | Greator than or equal to | 5 >= 2 | True |
<= | Less than or equal to | 5 <= 2 | False |
Here the output is:
a + b = 1
a - b = 0
a * b = 1156
a / b = 1
03) Logical operators
Operators | Description | Examples | Results |
---|---|---|---|
(&&) | It returns true when both operators are true or 1 | (5<2&&5>3)2> | False |
|| | It returns true when either operator is true or 1. | (5<2||5>3)2> | True |
! | It is used to reverse the logical state of the operand. | !(5<2) | True |
Here the output is:
a or b = 0
a or b = 1
04)Bitwise operators
To perform bit level operations, bitwise operators are used. They convert the values we provide to them in binary format.
a | b | a & b | a | b | a ^ b |
---|---|---|---|---|
0 | 0 | 0 | 0 | 0 |
0 | 1 | 0 | 1 | 1 |
1 | 1 | 1 | 1 | 0 |
1 | 0 | 0 | 1 | 1 |
Other Bitwise Operators
Symbols | Operators |
---|---|
(&) | Bitwise AND |
| | Bitwise OR |
^ | Bitwise XOR |
~ | Bitwise complement |
(<<) | Shift left |
(>>) | Shift right |
Here the output is: 2
05)Assignment operators
Operators | Description |
---|---|
= | Assigns values from right side operands to left side operand |
+= | It adds the right operand to the left operand and assign the result to the left operand. |
-= | It subtracts the right operand from the left operand and assigns the result to the left operand. |
*= | It multiplies the right operand with the left operand and assigns the result to the left operand. |
/= | It divides the left operand with the right operand and assigns the result to the left operand. |
Here the output is:
Equal to (=) is the assignment operator here, assigning 0 to a and 1 to b.
C Format Specifiers and Escape Sequences With Examples
Format Specifiers
The format specifier in C programming is used for input and output purposes. Through this, we tell the compiler what type of variable we are using for input using scanf() or printing using printf(). Some examples are %d, %c, %f, etc.
The %c and %d used in the printf( ) are called format specifiers. Format specifier tells printf( ) to print the value, for %c, a character will printed, and for %d, a decimal will be printed.Here is a list of format specifiers.
Format Specifier | Type |
---|---|
%c | Used to print the character |
%d | Used to print the signed integer |
%f | Used to print the float values |
%i | Used to print the unsigned integer |
%l | Used to long integer |
%if | Used to print the double integer |
%lu | Used to print the unsigned int or unsigned long integer |
%s | Used to print the String |
%u | Used to print the unsigned integer |
Following are the few examples of format specifier:
`star`To print the integer value:
`star`To print the float value:
`star`To print the character:
`star` To print the string:
What is Escape Sequence in C?
Many programming languages supports the concept of Escape Sequence. An escape sequence is a sequence of characters which are used in formatting the output. They are not displayed on the screen while printing. Each character has its own specific function like \t is used to insert a tab and \n is used to add newline.
Types of Escape Sequence in C
Escape Sequence | Description |
---|---|
\t | Inserts a tab |
\b | Inserts a backspace |
\n | Inserts a newline |
\r | Inserts a carriage return. |
\f | Inserts a form feed |
\' | Inserts a single quote character |
\'' | Inserts a double quote character |
\\ | Inserts a backslash character |
Following are the some examples of escape sequence:
`star`Print character backslash(\) using printf function
`star`Prints a newline before and after the text
`star`Use \" to print double quote and \' for single quote
`star`To provide tab space between two words
`star`To add vertical tab character
Code as described/written in the Escape Sequence
0 Comments